https://medium.com/@DoorDash/avoiding-cache-stampede-at-doordash-55bbf596d94b
Avoiding cache stampede at DoorDash
By Zohaib Sibte Hassan, Software Engineer at DoorDash
medium.com
DoorDash Cache 전략.
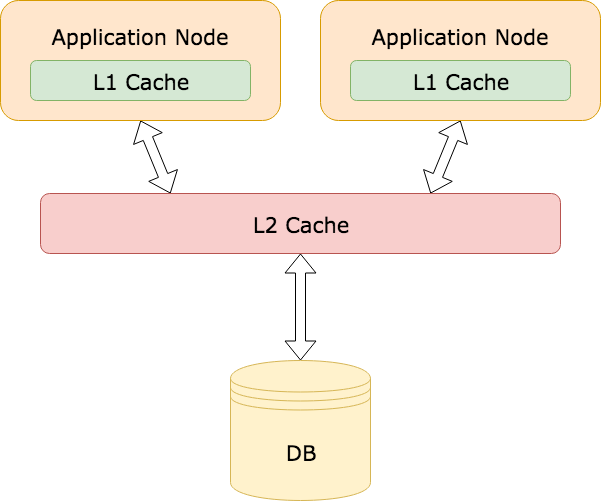
A typical caching setup
The debouncer approach
To solve the problem, we took inspiration from something front-end engineers use frequently. Debouncing is a common practice in the JS world to prevent duplicate events from firing and causing noise in the system. A well-known way to create a debouncing function looks something like this (using lodash or underscore.js):
https://github.com/syesildag/debounce/blob/master/src/main/java/debounce/DebounceExecutor.java
GitHub - syesildag/debounce
Contribute to syesildag/debounce development by creating an account on GitHub.
github.com
import java.util.concurrent.Callable;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.ThreadFactory;
import java.util.concurrent.TimeUnit;
import java.lang.Thread;
public class DebounceExecutor
{
private ScheduledExecutorService executor;
private ScheduledFuture<Object> future;
public DebounceExecutor()
{
this.executor = Executors.newSingleThreadScheduledExecutor(new ThreadFactory()
{
@Override
public Thread newThread(Runnable r)
{
Thread t = new Thread(r, "Debouncer");
t.setDaemon(true);
return t;
}
});
}
public ScheduledFuture<Object> debounce(long delay, Callable<Object> task)
{
if (this.future != null && !this.future.isDone())
this.future.cancel(false);
return this.future = this.executor.schedule(task, delay, TimeUnit.MILLISECONDS);
}
}
'emotional developer > detect-pattern' 카테고리의 다른 글
Five API Performance Optimization Tricks that Every Java Developer Must Know (0) | 2023.06.10 |
---|---|
System Design — Design A Rate Limiter (0) | 2023.05.19 |
5 Important Microservices Design Patterns (0) | 2023.05.19 |